Get Started with Docker
部署好難,一起來學 Docker!本篇文章會依序介紹使用 Docker 的理由、Docker 與 VM 的差別、一些基本指令以及如何撰寫 Dockerfile。有興趣的話就一起看下去吧!
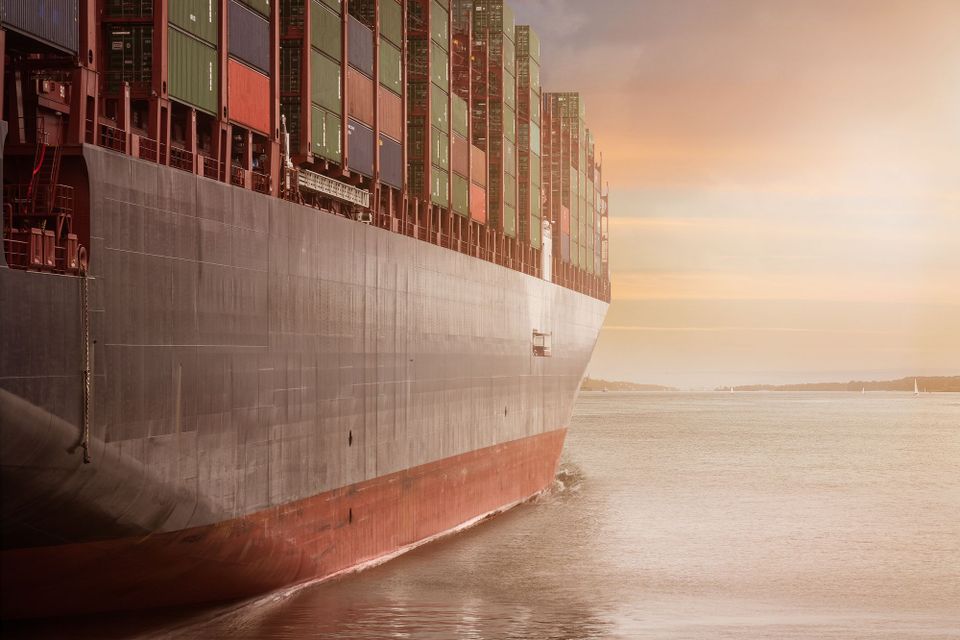
Why Docker
Problem (before containers)
- lots of individual machines or VMs
- hard to maintain the machines
- have to install application code on machines
Solution (Docker to the rescue)
Docker bundle all the application code, support binaries, and configuration together and pull them in image (only do once).
- build image: consistently package everything out
- ship image: easily ship these images to where you want
- run image: easily and consistently execute the applications
- CI/CD: being able to run the applications easily and test them in different environments
- different versions: easily run different versions of application without installing
- roll forward: when a defect is found, developers are able to fix it and deploy that new fix (don't have to pull it back)
Docker vs. VM
Docker
- have tiny containers run on the machine
- containers share a kernal
VM
- each VM has its own operating system (has their own kernal)
- running apps on those operating system
Basic Commands
Manage containers
Run a command in a new container
$ docker container run -it -p [local machine port]:[container exposed port] [image]
$ docker container run -d -p [local machine port]:[container exposed port] --name [NAME] [image]
-it
: interactive mode (run in the foreground)-d
: detached (run in the background)
List containers
$ docker container ls
$ docker container ls -a
// a shorter way
$ docker ps
$ docker ps -a
-a
: all
Remove one or more containers
$ docker container rm [CONTAINER ID]
// remove a running container
$ docker container rm [CONTAINER ID] -f
$ docker rm $(docker ps -aq) -f
-f
: force
Stop one or more running containers
$ docker container stop [CONTAINER ID]
Manage images
List images
$ docker images
Remove one or more images
$ docker image rm [IMAGE ID]
Pull an image or a repository from a registry
$ docker pull [image]
Run a command in a running container
$ docker container exec -it [NAME] bash
Writing Dockerfile
以 Python 為例
FROM python:3.8-slim-buster
WORKDIR /app
ENV PORT 8080
COPY requirements.txt requirements.txt
RUN pip3 install -r requirements.txt
COPY . .
CMD [ "python3", "main.py" ]
以 Node.js 為例
FROM node:17.0.1-alpine
WORKDIR /app
COPY . .
RUN npm i
EXPOSE 3000
CMD ["npm", "run", "start"]
FROM
: Set the baseImage to use for subsequent instructions.FROM
must be the first instruction in a Dockerfile.WORKDIR
: Set the working directoryENV
: Set the environment variable key to the value.COPY
: Copy files or folders from source to the dest path in the image's filesystem.RUN
: Execute any commands on top of the current image as a new layer and commit the results.EXPOSE
: Define the network ports that this container will listen on at runtime.CMD
: Provide defaults for an executing container.