不可不知的 JavaScript 基本觀念
今天要來介紹面前端工程師一定會被問的 JavaScript 基本觀念!var、let、const 有什麼差異?什麼時候使用雙等於 (==),什麼時候使用三等於 (===)?
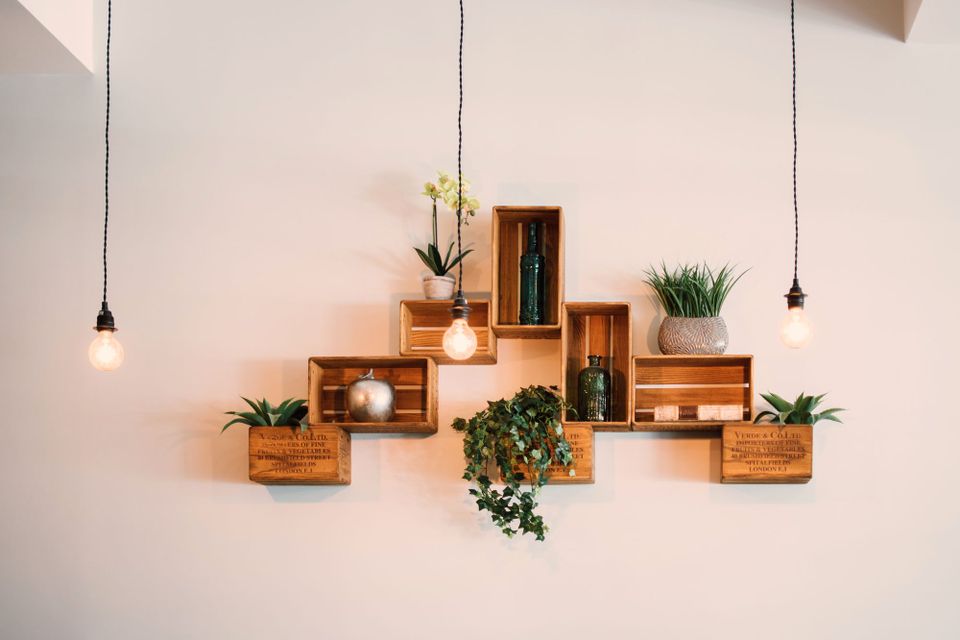
Variables vs. Let vs. Const
var
- can be redeclared
var x = "Joanne Tang";
var x = 21;
- can be used before it is declared
carName = "Benz";
var carName;
- have global scope
{
var x = 2;
}
// x CAN be used here
- don't use it anymore
let
- cannot be redeclared
var x = "Joanne Tang";
var x = 21;
// SyntaxError: 'x' has already been declared
- must be declared before use
- can be reassigned
- have block scope
{
let x = 2;
}
// x can NOT be used here
const
- cannot be redeclared
- must be assigned a value when they are declared
// Incorrect
const PI;
PI = 3.141592653589793;
- cannot be reassigned
const PI = 3.141592653589793;
PI = 3.14; // This will give an error
PI = PI + 10; // This will also give an error
- can change the elements of constant array
// You can create a constant array:
const cars = ["Benz", "Volvo", "BMW"];
// You can change an element:
cars[0] = "LEXUS";
// You can add an element:
cars.push("Audi");
const cars = ["Benz", "Volvo", "BMW"];
cars = ["LEXUS", "Volvo", "Audi"]; // ERROR
- can change the properties of constant object
// You can create a const object:
const person = {name:"Joanne Tang", age:"21", email:"[email protected]"};
// You can change a property:
person.email = "[email protected]";
// You can add a property:
person.gender = "female";
const person = {name:"Joanne Tang", age:"21", email:"[email protected]"};
person = {name:"Ben Wu", age:"25", email:"[email protected]"}; // ERROR
- have block scope
const x = 10;
// Here x is 10
{
const x = 2;
// Here x is 2
}
// Here x is 10
Comparision Operators
Operator | Description |
---|---|
== | equal value |
=== | equal value and type |
!= | not equal value |
!== | not equal value or type |